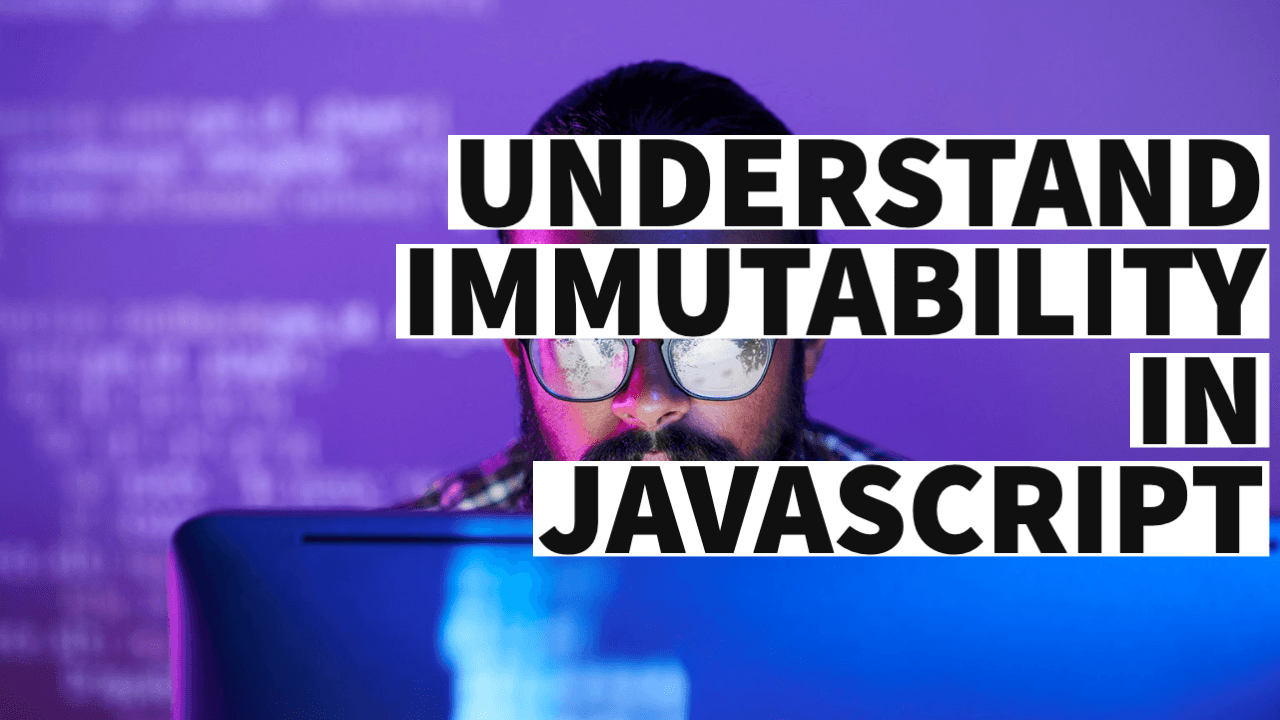
what is immutability in javascript
In JavaScript, immutability refers to the concept of an object or value that cannot be modified once it is created. It means that any attempt to change the state of an immutable object will result in the creation of a new object with the desired changes, rather than modifying the existing object directly.
Immutability is an important concept in JavaScript because it helps ensure the predictability and reliability of code. By using immutable data structures, you can avoid unexpected side effects and make it easier to reason about the behavior of your code.
In JavaScript, some data types are inherently immutable, such as strings and numbers. Once you create a string or a number, you cannot change its value. For example:
let name = "John";
name = "Jane"; // This creates a new string with the value "Jane", the original string "John" remains unchanged
However, objects and arrays in JavaScript are mutable by default. You can modify their properties or elements directly, which can sometimes lead to unintended consequences. To enforce immutability with objects and arrays, you can use various techniques such as:
- Object.freeze(): The
Object.freeze()
method prevents any modifications to an object, including adding or removing properties or changing their values.
const person = { name: "John", age: 30 };
Object.freeze(person);
person.name = "Jane"; // This change will be ignored in strict mode, and an error will be thrown in non-strict mode
- Immutable libraries: There are several third-party libraries available, such as Immutable.js and Immer, that provide data structures and utilities for working with immutable data in JavaScript.
import { Map } from 'immutable';
const person = Map({ name: "John", age: 30 });
const updatedPerson = person.set('name', 'Jane'); // Creates a new Map with the updated value
console.log(person.get('name')); // Output: "John"
console.log(updatedPerson.get('name')); // Output: "Jane"
By embracing immutability, you can write more robust and maintainable code in JavaScript by minimizing unintended side effects and making it easier to reason about state changes.
Certainly! Here are a few examples that demonstrate immutability in JavaScript:
- Immutable Strings:
let name = "John";
let newName = name + " Doe"; // Concatenating strings creates a new string
console.log(name); // Output: "John"
console.log(newName); // Output: "John Doe"
- Immutable Numbers:
let count = 5;
let newCount = count + 1; // Performing arithmetic operations creates a new number
console.log(count); // Output: 5
console.log(newCount); // Output: 6
- Immutable Arrays using Array methods:
let numbers = [1, 2, 3];
let newNumbers = numbers.concat(4); // Concatenating arrays creates a new array
console.log(numbers); // Output: [1, 2, 3]
console.log(newNumbers); // Output: [1, 2, 3, 4]
- Immutable Arrays using spread operator:
let numbers = [1, 2, 3];
let newNumbers = [...numbers, 4]; // Creating a new array using the spread operator
console.log(numbers); // Output: [1, 2, 3]
console.log(newNumbers); // Output: [1, 2, 3, 4]
- Immutable Objects using Object.assign():
let person = { name: "John", age: 30 };
let newPerson = Object.assign({}, person, { age: 31 }); // Creating a new object using Object.assign()
console.log(person); // Output: { name: "John", age: 30 }
console.log(newPerson); // Output: { name: "John", age: 31 }
- Immutable Objects using the spread operator:
let person = { name: "John", age: 30 };
let newPerson = { ...person, age: 31 }; // Creating a new object using the spread operator
console.log(person); // Output: { name: "John", age: 30 }
console.log(newPerson); // Output: { name: "John", age: 31 }
javascript different operation to perform immutable execution
In all of these examples, when we perform operations or create new objects or arrays, we are not modifying the original values. Instead, we are creating new instances with the desired changes, while the original values remain unchanged. This is the essence of immutability in JavaScript.
To perform immutable operations in JavaScript, there are several techniques and methods you can use. Here are some common operations and their corresponding immutable alternatives:
- Adding or Removing Elements in Arrays:
- Immutable Alternative: Use array methods like
concat()
,slice()
, or the spread operator (...
) to create a new array with the desired changes.
const numbers = [1, 2, 3];
const newNumbers = numbers.concat(4); // Adding an element
console.log(newNumbers); // Output: [1, 2, 3, 4]
const removedNumbers = numbers.slice(1); // Removing elements
console.log(removedNumbers); // Output: [2, 3]
const newNumbers = [...numbers, 4]; // Using the spread operator
console.log(newNumbers); // Output: [1, 2, 3, 4]
- Modifying Object Properties:
- Immutable Alternative: Use methods like
Object.assign()
, spread operator (...
), or libraries like Immer to create a new object with the desired changes.
const person = { name: "John", age: 30 };
const newPerson = Object.assign({}, person, { age: 31 }); // Modifying a property
console.log(newPerson); // Output: { name: "John", age: 31 }
const newPerson = { ...person, age: 31 }; // Using the spread operator
console.log(newPerson); // Output: { name: "John", age: 31 }
// Using Immer library
import produce from 'immer';
const newPerson = produce(person, draft => {
draft.age = 31;
});
console.log(newPerson); // Output: { name: "John", age: 31 }
- Modifying Nested Objects:
- Immutable Alternative: Use techniques like object spread, cloning nested objects, or libraries like Immer to create new objects with the desired changes.
const person = {
name: "John",
address: {
city: "New York",
country: "USA"
}
};
const newPerson = {
...person,
address: {
...person.address,
city: "San Francisco"
}
};
console.log(newPerson); // Output: { name: "John", address: { city: "San Francisco", country: "USA" } }
// Using Immer library
import produce from 'immer';
const newPerson = produce(person, draft => {
draft.address.city = "San Francisco";
});
console.log(newPerson); // Output: { name: "John", address: { city: "San Francisco", country: "USA" } }
By utilizing these techniques and methods, you can work with immutable data structures and ensure that the original values remain unchanged while creating new instances with the desired modifications.
immutability library in javascript
There are several libraries available in JavaScript that provide utilities and data structures for working with immutability. Here are some popular immutability libraries:
- Immutable.js: Immutable.js is a widely used library developed by Facebook that provides immutable data structures such as List, Map, and Set. It offers a rich set of methods for performing immutable operations on these data structures. Immutable.js uses structural sharing to efficiently create new versions of data without unnecessary copying.
Example usage of Immutable.js:
import { List, Map } from 'immutable';
const list1 = List([1, 2, 3]);
const list2 = list1.push(4); // Creates a new List with the added element
console.log(list1); // Output: List [1, 2, 3]
console.log(list2); // Output: List [1, 2, 3, 4]
const map1 = Map({ name: 'John', age: 30 });
const map2 = map1.set('age', 31); // Creates a new Map with the modified value
console.log(map1); // Output: Map { "name": "John", "age": 30 }
console.log(map2); // Output: Map { "name": "John", "age": 31 }
- Immer: Immer is a lightweight library that allows you to work with immutable data in a more convenient way. It provides a simple API for creating immutable updates to objects and arrays using a mutable syntax. Immer uses a technique called “copy-on-write” to ensure efficient updates without modifying the original data.
Example usage of Immer:
import produce from 'immer';
const person = { name: 'John', age: 30 };
const newPerson = produce(person, draft => {
draft.age = 31; // Modifying the draft object
});
console.log(person); // Output: { name: 'John', age: 30 }
console.log(newPerson); // Output: { name: 'John', age: 31 }
- Seamless-immutable: Seamless-immutable is another library that provides a simple API for creating and working with immutable data structures. It wraps JavaScript objects and arrays to ensure immutability. Seamless-immutable uses structural sharing to efficiently create new versions of data.
Example usage of Seamless-immutable:
import Immutable from 'seamless-immutable';
const data = Immutable({ name: 'John', age: 30 });
const newData = data.set('age', 31); // Creates a new Immutable object with the modified value
console.log(data); // Output: { name: 'John', age: 30 }
console.log(newData); // Output: { name: 'John', age: 31 }
These are just a few examples of immutability libraries available in JavaScript. Each library has its own features and API, so you can choose the one that best fits your project requirements and coding style.