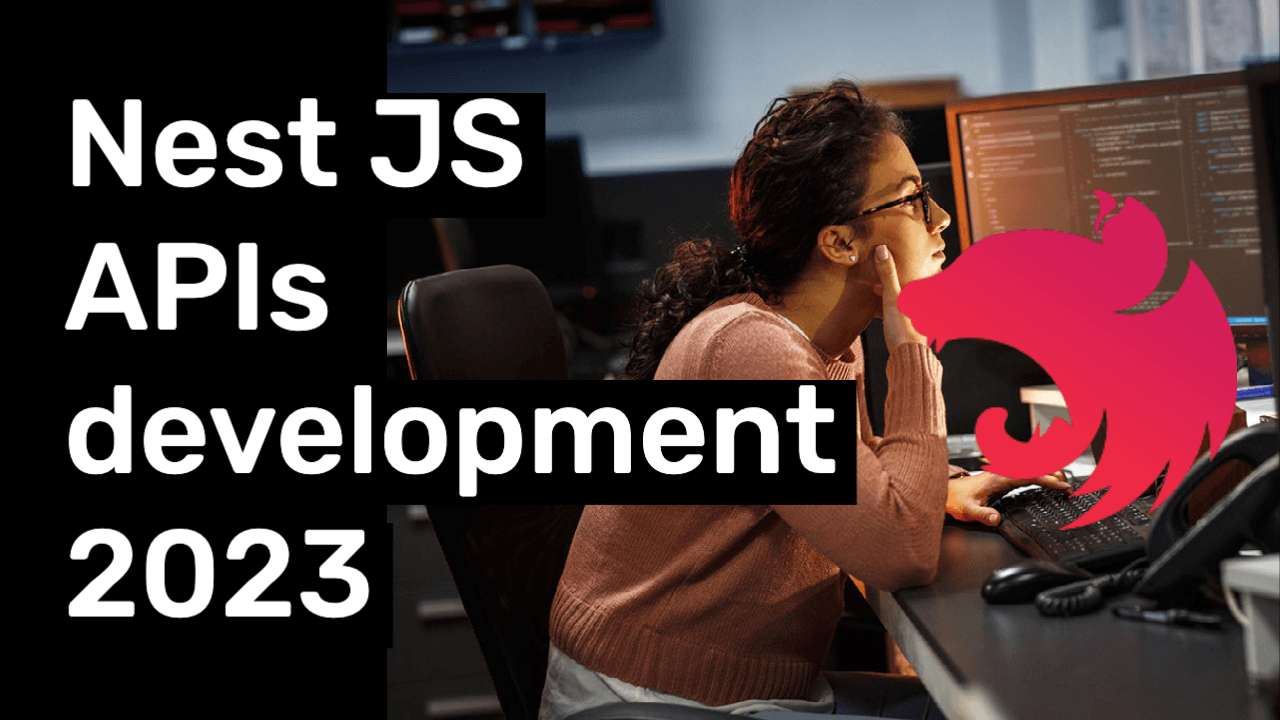
Nest JS Application using fastify adaptor
Github Link https://github.com/tkssharma/blogs/tree/master/nestjs-rest-apis-docs
In this post, I will demonstrate how to kickstart a simple RESTful APIs with NestJS from a newbie’s viewpoint.
What is NestJS?
As described in the Nestjs website, Nestjs is a progressive Node.js framework for building efficient, reliable and scalable server-side applications.
Nestjs combines the best programming practice and the cutting-edge techniques from the NodeJS communities.
- A lot of NestJS concepts are heavily inspired by the effort of the popular frameworks in the world, esp. Angular .
- Nestjs hides the complexities of web programming in NodeJS, it provides a common abstraction of the web request handling, you are free to choose Expressjs or Fastify as the background engine.
- Nestjs provides a lot of third party project integrations, from database operations, such as Mongoose, TypeORM, etc. to Message Brokers, such as Redis, RabbitMQ, etc.
If you are new to Nestjs like me but has some experience of Angular , TypeDI or Spring WebMVC, bootstraping a Nestjs project is really a piece of cake.
Generating a NestJS project
Make sure you have installed the latest Nodejs.
npm i -g @nestjs/cli
When it is finished, there is a nest
command available in the Path
. The usage of nest
is similar with ng
(Angular CLI), type nest --help
in the terminal to list help for all commands.
❯ nest --help
Usage: nest <command> [options]
Options:
-v, --version Output the current version.
-h, --help Output usage information.
Commands:
new|n [options] [name] Generate Nest application.
build [options] [app] Build Nest application.
start [options] [app] Run Nest application.
info|i Display Nest project details.
update|u [options] Update Nest dependencies.
add [options] <library> Adds support for an external library to your project.
generate|g [options] <schematic> [name] [path] Generate a Nest element.
Available schematics:
┌───────────────┬─────────────┐
│ name │ alias │
│ application │ application │
│ class │ cl │
│ configuration │ config │
│ controller │ co │
│ decorator │ d │
│ filter │ f │
│ gateway │ ga │
│ guard │ gu │
│ interceptor │ in │
│ interface │ interface │
│ middleware │ mi │
│ module │ mo │
│ pipe │ pi │
│ provider │ pr │
│ resolver │ r │
│ service │ s │
│ library │ lib │
│ sub-app │ app │
└───────────────┴─────────────┘
Now generate a Nestjs project via:
nest new nestjs-sample
Open it in your favorite IDEs, such as Intellij WebStorm or VSCode.
Exploring the project files
Expand the project root, you will see the following like tree nodes.
.
├── LICENSE
├── nest-cli.json
├── package.json
├── package-lock.json
├── README.md
├── src
│ ├── app.controller.spec.ts
│ ├── app.controller.ts
│ ├── app.module.ts
│ ├── app.service.ts
│ └── main.ts
├── test
│ ├── app.e2e-spec.ts
│ └── jest-e2e.json
├── tsconfig.build.json
└── tsconfig.json
The default structure of this project is very similar with the one generated by Angular CLI.
- src/main.ts is the entry file of this application.
- src/app* is the top level component in a nest application.
- There is an app.module.ts is a Nestjs
Module
which is similar with AngularNgModule
, and used to organize codes in the logic view. - The app.service.ts is an
@Injectable
component, similar with the service in Angular or Spring’s Service, it is used for handling business logic. A service is annotated with@Injectable
. - The app.controller.ts is the controller of MVC, to handle incoming request, and responds the handled result back to client. The annotatoin
@Controller()
is similar with Spring MVC’s@Controller
. - The app.controller.spec.ts is test file for app.controller.ts. Nestjs uses Jest as testing framework.
- There is an app.module.ts is a Nestjs
- test folder is for storing e2e test files.
Here’s a comparison between Express and Fastify frameworks
-
Performance: Fastify is known for its excellent performance. It is built with a focus on speed and claims to be one of the fastest web frameworks available. It achieves this by utilizing an efficient routing engine, optimized request/response processing, and low overhead. Express is also performant, but Fastify generally outperforms it in terms of raw speed and request handling.
-
Routing: Both Express and Fastify provide robust routing capabilities. Express has a flexible routing system that allows defining routes using various HTTP methods and URL patterns. It supports middleware functions and parameter parsing. Fastify, on the other hand, has a built-in and highly efficient routing engine that supports similar functionalities to Express. However, Fastify’s routing engine is optimized for speed and handles routes with great efficiency.
-
Middleware: Express has a vast ecosystem of middleware modules that allow developers to easily add functionality to their applications. It provides a simple middleware architecture that supports functions and middleware libraries. Fastify also supports middleware, but its ecosystem is not as extensive as Express. However, Fastify’s middleware execution is more efficient due to its design and architecture.
-
Extensibility: Express is known for its extensive ecosystem and a large number of available plugins and middleware. It has been around for a long time, and developers have built numerous extensions and integrations for it. Fastify, although not as mature as Express, has a growing ecosystem and offers a good selection of plugins. However, if you require specific integrations or have dependencies on certain plugins, Express may have a wider range of options available.
-
Community and Documentation: Express has a mature and well-established community with extensive documentation, tutorials, and resources available. It has been widely adopted and has a large user base. Fastify, being a relatively newer framework, has a smaller but growing community. While its documentation is good, it may not be as extensive as Express. However, Fastify’s documentation is concise, clear, and provides good examples.
-
Learning Curve: Express is considered beginner-friendly and has a gentle learning curve. It provides a simple and intuitive API, making it easy to get started. Fastify, although similar in some aspects, may have a steeper learning curve due to its advanced features and optimized design. However, if you’re familiar with Express, transitioning to Fastify should not be too difficult.
In summary, Fastify excels in terms of performance and is designed for speed and efficiency. It is a great choice for high-performance applications or situations where raw speed is a priority. Express, on the other hand, has a larger community, extensive ecosystem, and wider adoption. It is a solid choice for a wide range of applications and has a more gentle learning curve. Ultimately, the choice between Express and Fastify depends on your specific requirements, performance needs, ecosystem preferences, and familiarity with the frameworks.
Fastify adapter available for NestJS
there is no official Fastify adapter available for NestJS. However, NestJS provides built-in support for Express as its default HTTP server framework.
If you prefer to use Fastify as the HTTP server for your NestJS application, you can achieve that by creating a custom integration. Here are the general steps you can follow:
-
Install the required dependencies:
npm install fastify fastify-reply-from @nestjs/core reflect-metadata rxjs
-
Create a custom Fastify adapter file, let’s name it
fastify-adapter.ts
:import { INestApplication } from '@nestjs/common'; import { NestFactory } from '@nestjs/core'; import { FastifyAdapter, NestFastifyApplication } from '@nestjs/platform-fastify'; import { AppModule } from './app.module'; async function bootstrap() { const app = await NestFactory.create<NestFastifyApplication>(AppModule, new FastifyAdapter()); // Additional Fastify-specific configuration can be done here // app.use(...) await app.listen(3000); } bootstrap();
-
Create or modify your
main.ts
file to use the custom Fastify adapter:import { fastifyAdapter } from './fastify-adapter'; async function bootstrap() { const app = await NestFactory.create(AppModule, fastifyAdapter); await app.listen(3000); } bootstrap();
-
Modify the
package.json
file to include the necessary scripts:"scripts": { "start": "ts-node main.ts" }
With these steps, you can use Fastify as the HTTP server for your NestJS application. However, please note that this approach involves creating a custom integration and may require additional configuration or adjustments based on your specific needs. Be sure to consult the Fastify documentation for further details on configuring Fastify-specific features and plugins.
Using Fastify as the HTTP server adapter for NestJS offers several advantages:
-
Performance: Fastify is known for its exceptional performance. It is built with a focus on speed and efficiency, resulting in faster request/response processing compared to other HTTP server frameworks. By using Fastify as the adapter, you can leverage its performance benefits to enhance the overall speed and responsiveness of your NestJS application.
-
Low Overhead: Fastify has a low memory footprint and low resource utilization. It achieves this by utilizing a highly efficient routing engine and optimized request handling. By integrating Fastify into your NestJS application, you can benefit from its lightweight nature and reduce unnecessary overhead, allowing your application to handle a larger number of concurrent requests efficiently.
-
Ecosystem Compatibility: Although NestJS has a vibrant ecosystem and extensive support for Express, it may not have as many third-party packages or integrations specifically tailored for Fastify. However, by using Fastify as the adapter, you can still leverage the broad range of Fastify plugins, middlewares, and integrations available within the Fastify ecosystem. This provides you with additional options and flexibility when building your application.
-
Async/Await Support: Fastify natively supports async/await, which aligns well with NestJS’s focus on asynchronous programming. This compatibility simplifies the development process and allows you to write clean, readable, and expressive code using async/await syntax throughout your NestJS application.
-
TypeScript Support: Fastify has excellent TypeScript support, making it a seamless fit with NestJS, which is built with TypeScript. The type inference and autocompletion features of TypeScript can enhance your development experience, providing better tooling and reducing the likelihood of runtime errors.
-
Fastify-Specific Features: Fastify offers various features that can benefit your application, such as advanced request/response validation, request hooks, logging, and support for JSON Schema. By using Fastify as the adapter, you can take advantage of these features and tailor them to your specific use cases within your NestJS application.
It’s important to note that while using the Fastify adapter provides these advantages, it may require additional configuration and adjustment compared to the default Express adapter. Additionally, it’s recommended to carefully assess your specific application requirements, performance needs, and compatibility with the Fastify ecosystem before opting for the Fastify adapter in your NestJS application.