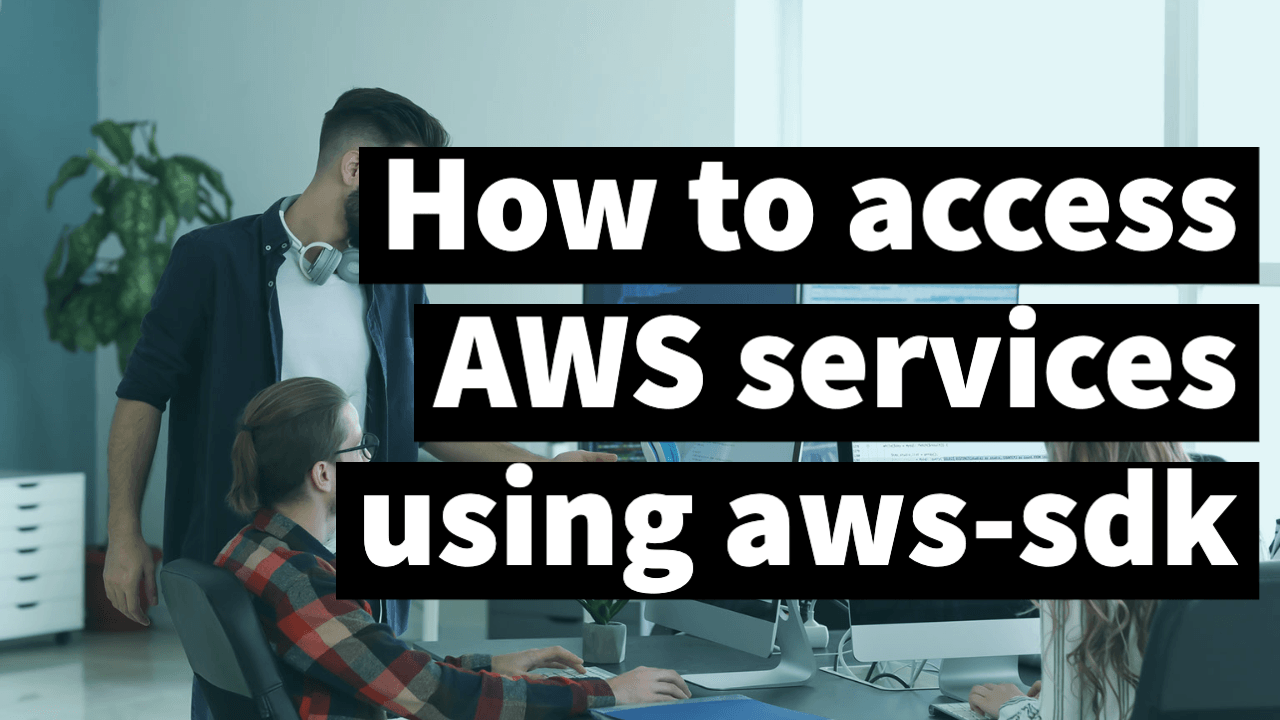
When should we use Neo4j
Neo4j, as a graph database, has several use cases where its graph-oriented data model and powerful querying capabilities provide significant advantages. Here are some common use cases for using Neo4j:
-
Social Networks: Neo4j is well-suited for modeling social network data. It can efficiently represent relationships between users, their connections, and their activities. With Neo4j, you can easily traverse and query the graph to retrieve social connections, analyze social interactions, recommend connections, and perform social network analysis.
-
Recommendation Engines: Neo4j is effective in building recommendation engines. By modeling user preferences, item attributes, and user-item relationships in the graph, you can leverage Neo4j’s graph algorithms and query capabilities to provide personalized recommendations based on similarities, graph traversals, and collaborative filtering techniques.
-
Fraud Detection: Neo4j’s graph data model is beneficial for fraud detection and prevention. By representing entities (e.g., users, transactions, devices) as nodes and their relationships as edges, Neo4j enables the detection of suspicious patterns, network analysis, and anomaly detection. It allows you to uncover hidden connections, identify fraudulent behavior, and prevent financial and cybersecurity threats.
-
Knowledge Graphs: Neo4j is often used to build knowledge graphs that organize and represent complex, interconnected information. With Neo4j, you can model entities, their attributes, and their relationships, enabling powerful semantic queries, efficient navigation of the graph, and the ability to explore and analyze knowledge domains.
-
Recommendation Systems: Neo4j can power recommendation systems by modeling user preferences, item characteristics, and user-item interactions. Its graph-based approach enables collaborative filtering, content-based recommendations, and hybrid recommendation strategies. Neo4j’s ability to capture and traverse complex relationships makes it suitable for building sophisticated recommendation algorithms.
-
Network and IT Operations: Neo4j is used for network and IT operations management. It allows you to model network topologies, dependencies between systems, and IT infrastructure components. By leveraging Neo4j’s graph algorithms and querying capabilities, you can gain insights into network performance, detect bottlenecks, troubleshoot issues, and optimize network operations.
-
Bioinformatics and Life Sciences: Neo4j is employed in bioinformatics and life sciences research. It helps in modeling biological networks, gene interactions, protein-protein interactions, and complex relationships within biological systems. Neo4j’s graph-based approach enables efficient analysis, pattern recognition, and visualization of biological data.
-
Content Management: Neo4j can be used for content management systems. By modeling content entities (e.g., articles, authors, categories) and their relationships, Neo4j allows efficient content retrieval, categorization, and recommendation. It helps in building intelligent content recommendation engines, personalized content delivery, and content discovery platforms.
These are just a few examples of the diverse use cases where Neo4j can provide significant benefits. Its ability to handle complex relationships, perform graph traversals, and execute powerful graph queries makes it a powerful tool for a wide range of applications that involve connected data.
what is neo4j and how to work with neo4j
Neo4j is a highly scalable, graph database management system (DBMS) that is designed to store, manage, and query graph data. It is based on the property graph model, which represents data as nodes, relationships, and properties. Nodes represent entities or objects, relationships represent the connections between nodes, and properties provide additional information about nodes and relationships.
Here’s an overview of how to work with Neo4j:
-
Data Modeling: Start by defining your graph data model. Identify the entities you want to represent as nodes and the relationships between them. Determine the properties that describe each node and relationship.
-
Neo4j Browser: Neo4j provides a web-based interface called the Neo4j Browser, which allows you to interact with the database using the Cypher query language. You can access the browser by visiting http://localhost:7474/ after starting the Neo4j server.
-
Cypher Query Language: Cypher is a declarative query language specifically designed for querying and manipulating graph data in Neo4j. It uses ASCII-art syntax to express patterns and operations on the graph. You can use Cypher to create, read, update, and delete nodes and relationships, as well as perform complex graph queries.
-
Neo4j Drivers: Neo4j provides official drivers for various programming languages, including Node.js, Python, Java, and more. These drivers allow you to connect to the Neo4j database from your applications and execute Cypher queries programmatically.
-
Connect to Neo4j: To work with Neo4j in your application, you need to establish a connection to the database using the appropriate Neo4j driver for your programming language. Once connected, you can execute Cypher queries and retrieve the results.
-
Querying Graph Data: With Neo4j, you can perform powerful graph queries to traverse the relationships and retrieve specific patterns from the graph. Cypher allows you to filter, sort, and aggregate data, as well as perform graph algorithms and text search.
-
Indexing and Constraints: Neo4j allows you to define indexes and constraints on your graph data to optimize query performance and ensure data integrity. Indexes speed up queries by allowing you to quickly locate nodes based on certain properties, while constraints enforce rules to maintain the integrity of your data.
-
Transactions: Neo4j supports ACID (Atomicity, Consistency, Isolation, Durability) transactions, ensuring data integrity and consistency. You can group multiple database operations into a single transaction to guarantee that either all the operations succeed or none of them are applied.
-
Visualization: Neo4j provides various visualization tools, such as the Neo4j Browser, Neo4j Bloom, and integrations with third-party visualization libraries, to help you visualize and explore your graph data.
Working with Neo4j involves understanding graph data modeling, using the Cypher query language, connecting to the database with drivers, executing queries, and leveraging the features and capabilities of Neo4j to efficiently store and retrieve graph data.
How To set up Neo4j with Node.js, you can follow these steps:
-
Install Neo4j: Download and install Neo4j from the official website (https://neo4j.com/download/). Choose the appropriate version for your operating system and follow the installation instructions.
-
Set up a Neo4j database: Launch the Neo4j server and create a new database instance. You can access the Neo4j browser by visiting http://localhost:7474/ in your web browser. Follow the instructions to create a new database and set a username and password.
-
Create a Node.js project: Set up a new Node.js project by creating a new directory and running
npm init
in the terminal. This will create apackage.json
file for your project. -
Install the Neo4j driver: Install the official Neo4j driver for Node.js by running the following command in your project directory:
npm install neo4j
-
Import the Neo4j driver: In your Node.js project, import the Neo4j driver by adding the following line at the top of your JavaScript file:
const neo4j = require('neo4j');
-
Connect to the Neo4j database: Create a new instance of the Neo4j driver and establish a connection to your Neo4j database using the following code:
const driver = neo4j.driver('bolt://localhost', neo4j.auth.basic('username', 'password')); const session = driver.session();
Replace
'username'
and'password'
with the credentials you set up during the database creation. -
Run Cypher queries: You can now execute Cypher queries against the Neo4j database using the
session.run()
method. Here’s an example that retrieves all nodes in the database:session.run('MATCH (n) RETURN n') .then(result => { result.records.forEach(record => { console.log(record.get('n').properties); }); session.close(); }) .catch(error => { console.error(error); });
Customize the Cypher query according to your needs.
-
Close the connection: Once you’re done with the database operations, close the connection by calling
session.close()
anddriver.close()
to release the resources:session.close(); driver.close();
That’s it! You have successfully set up Neo4j with Node.js. You can now integrate Neo4j into your Node.js application and perform various database operations using the Neo4j driver.
Simple example with Neo4j and express
To set up APIs that access data from Neo4j in Node.js, you can follow these steps:
-
Install necessary dependencies: Start by creating a new Node.js project or navigating to your existing project. In your project directory, run the following command to install the required dependencies:
npm install express neo4j
This installs the Express framework and the Neo4j driver for Node.js.
-
Create an Express server: Set up an Express server in your Node.js project by creating a new JavaScript file (e.g.,
server.js
). Import the necessary modules and create an instance of the Express application:const express = require('express'); const app = express(); // Additional code...
-
Connect to Neo4j: Import the Neo4j driver and establish a connection to your Neo4j database as mentioned earlier in the previous response:
const neo4j = require('neo4j'); const driver = neo4j.driver('bolt://localhost', neo4j.auth.basic('username', 'password')); const session = driver.session(); // Additional code...
-
Define API endpoints: Create the necessary API endpoints to access and manipulate data from Neo4j. For example, you can define a route to retrieve all nodes from the database:
app.get('/nodes', (req, res) => { session.run('MATCH (n) RETURN n') .then(result => { const nodes = result.records.map(record => record.get('n').properties); res.json(nodes); }) .catch(error => { res.status(500).json({ error: error.message }); }); }); // Additional endpoints...
Customize the Cypher query and define other endpoints based on your application’s requirements.
-
Start the server: Add the following code to start the Express server and listen for incoming requests on a specific port (e.g., port 3000):
const port = 3000; app.listen(port, () => { console.log(`Server is listening on port ${port}`); });
-
Test the APIs: Run the Node.js application by executing the command
node server.js
in your project directory. You can now access the defined API endpoints (e.g.,http://localhost:3000/nodes
) to retrieve data from Neo4j.
Remember to handle error cases appropriately, such as when the connection to Neo4j fails or when the Cypher query encounters an error. You can customize the code to handle different scenarios based on your application’s needs.
That’s it! You have set up APIs in Node.js that can access data from Neo4j. You can expand on this foundation to define more complex API endpoints, perform data manipulation operations, and integrate Neo4j into your application’s data access layer.
neo4J with Nest JS example impl
To use Neo4j with NestJS, you can follow these steps:
-
Set up a new NestJS project: Create a new NestJS project using the NestJS CLI or by cloning a boilerplate project. You can install the NestJS CLI globally by running the following command:
npm install -g @nestjs/cli
Then, generate a new NestJS project by running:
nest new project-name
Navigate to the project directory:
cd project-name
-
Install necessary dependencies: In your project directory, install the required dependencies by running the following command:
npm install @nestjs/graphql apollo-server-express neo4j-driver
This installs the necessary packages for using GraphQL, Apollo Server with Express, and the Neo4j driver.
-
Set up Neo4j configuration: Create a
neo4j.config.ts
file in the project’ssrc/config
directory. In this file, define the Neo4j configuration using the Neo4j driver:import { ConfigModule, ConfigService } from '@nestjs/config'; import { Neo4jModuleOptions } from 'neo4j-driver'; export const neo4jConfig: Neo4jModuleOptions = { scheme: 'neo4j', host: 'localhost', port: 7687, username: 'your-username', password: 'your-password', };
Replace
'your-username'
and'your-password'
with the appropriate Neo4j credentials. -
Configure the Neo4j module: Open the
src/app.module.ts
file and import the necessary modules:import { Module } from '@nestjs/common'; import { ConfigModule, ConfigService } from '@nestjs/config'; import { Neo4jModule } from 'nest-neo4j'; @Module({ imports: [ ConfigModule.forRoot(), Neo4jModule.forRootAsync({ imports: [ConfigModule], useFactory: async (configService: ConfigService) => ({ uri: configService.get<string>('neo4j.uri'), username: configService.get<string>('neo4j.username'), password: configService.get<string>('neo4j.password'), }), inject: [ConfigService], }), ], }) export class AppModule {}
-
Create a GraphQL resolver: Generate a new GraphQL resolver using the NestJS CLI. Run the following command:
nest generate resolver
This will create a new resolver file in the
src
directory. -
Define GraphQL queries and mutations: Open the newly generated resolver file (
src/resolver.ts
) and define your GraphQL queries and mutations. Here’s an example:import { Resolver, Query, Mutation, Args } from '@nestjs/graphql'; import { Neo4j } from 'nest-neo4j'; @Resolver() export class MyResolver { constructor(private readonly neo4j: Neo4j) {} @Query() async getNodeById(@Args('id') id: string) { const result = await this.neo4j.query(` MATCH (n:Node {id: $id}) RETURN n `, { id }); return result.records.map(record => record.get('n')); } @Mutation() async createNode(@Args('input') input: any) { const result = await this.neo4j.query(` CREATE (n:Node $input) RETURN n `, { input });
return result.records.map(record => record.get(‘n’)); } }
Customize the Cypher queries and resolver methods based on your application's needs.
7. Create a GraphQL module: Open the `src/app.module.ts` file and import the necessary modules to enable GraphQL and provide the resolvers:
```typescript
import { Module } from '@nestjs/common';
import { GraphQLModule } from '@nestjs/graphql';
import { Neo4jModule } from 'nest-neo4j';
import { MyResolver } from './resolver';
@Module({
imports: [
ConfigModule.forRoot(),
Neo4jModule.forRootAsync({
imports: [ConfigModule],
useFactory: async (configService: ConfigService) => ({
uri: configService.get<string>('neo4j.uri'),
username: configService.get<string>('neo4j.username'),
password: configService.get<string>('neo4j.password'),
}),
inject: [ConfigService],
}),
GraphQLModule.forRoot({
autoSchemaFile: 'schema.gql',
}),
],
providers: [MyResolver],
})
export class AppModule {}
-
Generate a GraphQL schema: In the terminal, run the following command to generate the GraphQL schema file (
schema.gql
):nest generate schema
-
Start the application: Finally, start the NestJS application by running the following command:
npm run start:dev
This will start the application and expose the GraphQL endpoint.
That’s it! You have set up a NestJS application with Neo4j and GraphQL. You can now define and execute GraphQL queries and mutations that interact with the Neo4j database.